TransferNow API & SDK
to upload, share, and download large files
With just a few lines of code and in a matter of minutes, TransferNow allows developers to integrate a secure solution for transferring large files on websites, mobile apps, SaaS solutions, or any other custom workflow.
TransferNow API is used by companies all around the world
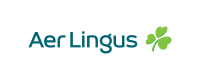
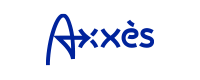
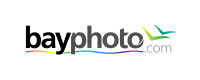
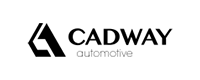
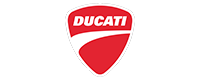
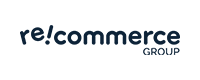
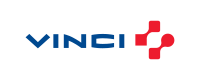
A powerful API for uploading files
Save valuable time
On a daily basis, we work to provide you with the best possible tool for transferring files securely.
You don't have to develop new systems or implement new features that can take months of work to upload files. TransferNow takes care of everything for you with our magic API!
Start sending files from your solution with our easy-to-integrate SDK. Our file transfer API addresses and covers all the needs and use cases of businesses of all sizes.
Pay for what you use
Try our solution for free for 14 days and get 100 GB of API storage included to integrate our API and perform your tests. No payment method is required to create an account.
Once your tests and integration are complete, the average number of gigabytes (GB) consumed will be billed to you at the beginning of the following month for the past period (US$ 0.2/GB/month). The rate per gigabyte (GB) decreases based on the volume consumed.
With Pay-As-You-Go billing, you get optimized storage capacity and most importantly, you save gigabytes!
We are also developers
We are developers too, and we know how many constraints there are for uploading and efficiently distributing numerous file transfers.
Save precious time by implementing the few lines of code that will allow you to upload and send files up to 5 TB per transfer in just a few minutes.
Join thousands of businesses
Thousands of businesses of all sizes, from startups to large enterprises, use TransferNow's software and APIs to securely upload and send large files daily.
Your files are secure
At TransferNow, there is no compromise when it comes to your data.
By using our service to upload and transfer files, you have access to a dedicated and secure global cloud infrastructure where files are stored and encrypted on disk (AES-XTS 256 bits) in datacenters (AICPA SOC 2 Type II) across the European, American, and Asian continents.
Use the SSE-C (Server-Side Encryption with Customer-Provided keys) feature to protect access to the download of your files and encrypt them on disk with your own key/passphrase.
An SDK to facilitate file upload and sharing
In a few minutes, integrate our SDK to securely upload and transfer files programmatically.
With our API, cover all uses and use cases, even the most complex ones!
Consult the documentationTransferNow API Features
Resilient multipart upload up to 5 TB per file
Upload local
Upload remote (URL)
Selection of the storage region
Files available for up to 365 days
Email notifications
Do you have a question?
Ready to get started?
Integrate our API/SDK – 5 minutes, a few lines of code.
14-day free trial – 100 GB of storage
Try for freeNo credit card required